App.All vs App.Use
When it comes to building applications using Node.js and Express, there are two important methods that developers commonly utilize: App.All and App.Use. These methods allow developers to handle different types of requests and define middleware functions. However, understanding the differences and use cases for each method is crucial in order to leverage their full potential.
Key Takeaways:
- App.All and App.Use are methods used in Node.js and Express for handling requests and defining middleware functions.
- App.All is used to handle all types of HTTP requests for a given route, while App.Use is used to define middleware that applies to all routes.
- Since App.All is route-specific, it should be used when you want to handle all types of HTTP requests for a particular route.
- App.Use is more general-purpose and can be used to define middleware that applies to all routes, regardless of their specific HTTP method.
Let’s take a closer look at these two methods and understand their differences in greater detail.
Understanding App.All
The App.All method in Express allows you to handle requests for a specific route regardless of their HTTP method. This means that if you define a route using app.all('/myroute', callback)
, the callback function will be executed for any type of request made to that route, whether it’s a GET, POST, PUT, DELETE, etc.
This flexibility provided by App.All makes it particularly useful when you want to perform the same operation, regardless of the HTTP method used.
Here’s a simple example to illustrate:
HTTP Method | Route | Response |
---|---|---|
GET | /myroute | Handle GET request for /myroute |
POST | /myroute | Handle POST request for /myroute |
In this example, defining the route as app.all('/myroute', callback)
would handle both GET and POST requests to /myroute
using the same callback function.
Understanding App.Use
The App.Use method, on the other hand, is used to define middleware functions that should be executed for all routes and all HTTP methods. It provides a way to apply common functionality or transformations to incoming requests or outgoing responses, regardless of the specific routes or methods being used.
Applying middleware using App.Use is a powerful way to centralize common functionality throughout your application.
For example, you might want to log the request details for every incoming request, or apply authentication logic to all routes. By using app.use(middleware)
, you can execute the middleware function for every request that comes into your application.
Comparing App.All and App.Use
To summarize the key differences between App.All and App.Use, we can use the following table:
App.All | App.Use |
---|---|
Handles requests for a specific route regardless of their HTTP method. | Applies middleware functions to all routes and all HTTP methods. |
Route-specific. | General-purpose. |
Particularly useful when performing the same operation for any type of request to a specific route. | Useful for applying common functionality or transformations to all incoming requests or outgoing responses. |
Conclusion
In conclusion, understanding when and how to use App.All and App.Use is essential for building efficient and maintainable applications with Node.js and Express. By leveraging the route-specific functionality of App.All and the general-purpose power of App.Use, developers can streamline their code and apply common functionality with ease, resulting in better overall application architecture and performance.
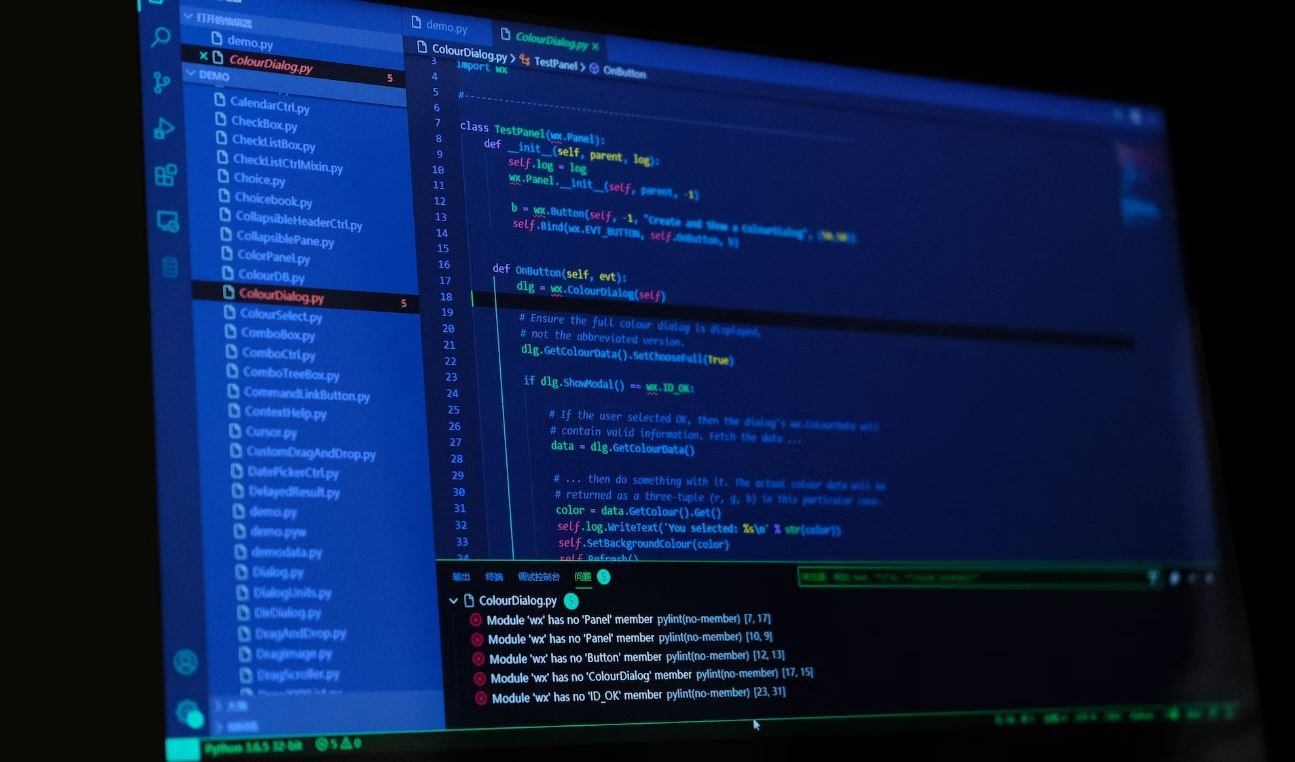
Common Misconceptions
People often have misconceptions about the difference between App.All and App.Use
One common misconception is that App.All and App.Use are interchangeable and can be used interchangeably in an application. However, this is not the case. App.All is used to register middleware globally for all requests, whereas App.Use is used to register middleware for a specific route or set of routes.
- App.All registers middleware for all requests.
- App.Use registers middleware for a specific route or set of routes.
- App.All is executed before App.Use middleware.
Another misconception is that App.All and App.Use have the same execution order.
While it may seem logical to assume that since App.All registers middleware globally and App.Use registers it for specific routes, they would have the same execution order. However, this is not the case. App.All middleware is executed before App.Use middleware, regardless of the order in which they are registered.
- App.All middleware is executed before App.Use middleware.
- The execution order of App.All and App.Use can be modified using the next() function within the middleware.
- Changing the execution order of App.All and App.Use can have implications on the functionality of the application.
It is also a common misconception that App.All middleware applies to all routes, including error routes.
Although App.All middleware is executed for all requests, including error routes, it does not apply to errors themselves. Error handling in an application requires separate middleware to handle them. App.All middleware cannot intercept and handle errors for the entire application.
- App.All middleware is executed for all requests, including error routes.
- Separate middleware is needed to handle errors in an application.
- App.All middleware cannot handle errors for the entire application.
Many people mistakenly believe that App.All and App.Use are unnecessary in an application.
Some developers may think that using App.All or App.Use middleware is unnecessary in their application, especially if they are not familiar with its purpose or the specific middleware they need. However, middleware plays a crucial role in handling requests, modifying responses, and implementing additional functionality that improves the overall performance, security, and user experience of an application.
- Middleware improves the performance, security, and user experience of an application.
- App.All and App.Use middleware provide flexibility and control over the request-handling process.
- Using appropriate middleware can enhance the functionality of an application.
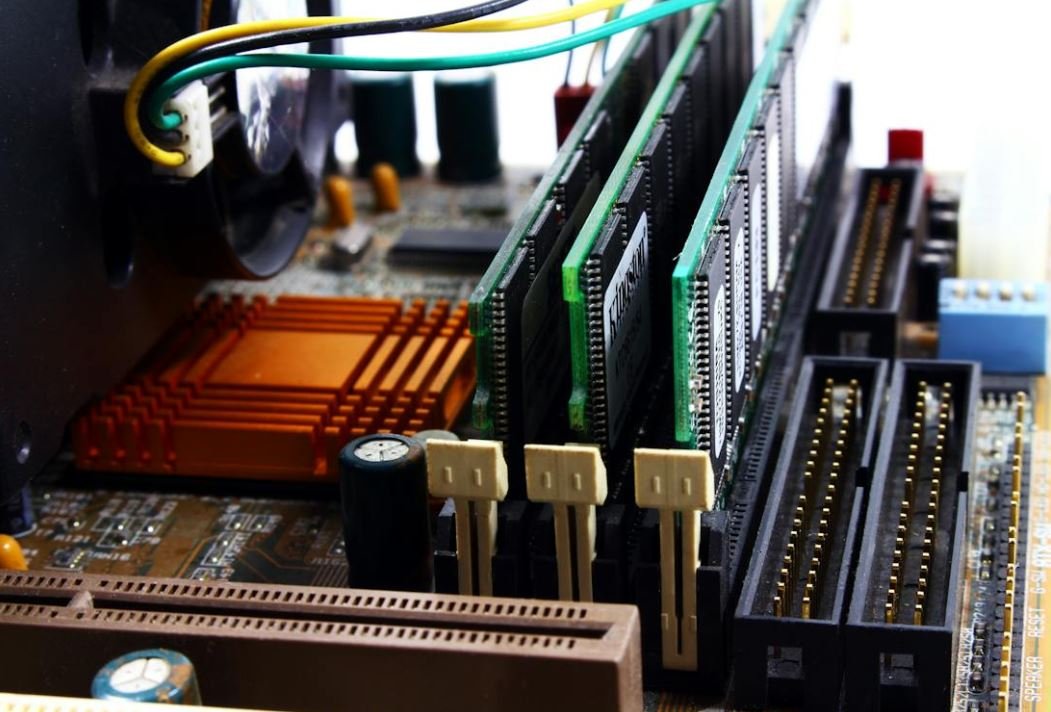
Introduction
App.All and App.Use are two common middleware functions in the world of web development. While both are used to handle request and response objects in Node.js, there are some key differences between them. In this article, we will examine these differences and explore the scenarios where one might be preferable over the other.
Table A: Comparison of Purpose
Table A provides an overview of the primary purpose of both App.All and App.Use middleware functions.
App.All Middleware | App.Use Middleware |
---|---|
Used to handle all types of HTTP requests | Used to handle specific HTTP requests |
Executes for all routes in the application | Executes for specific routes matching the defined path |
Commonly used for error handling and global middleware | Commonly used for route-specific middleware |
Table B: Scope of Effect
Table B illustrates the scope and extent of effect of both App.All and App.Use middleware functions.
App.All Middleware | App.Use Middleware |
---|---|
Executes for all requests, regardless of the request method | Executes only for requests with specific HTTP methods |
Affects the entire application | Affects only the routes it is applied to |
Can modify the request/response objects globally | Can modify the request/response objects for specific routes only |
Table C: Middleware Order
Middlewares are executed in a specific order. Table C displays the ordering of App.All and App.Use middlewares.
App.All Middleware | App.Use Middleware |
---|---|
Executed in the order they are defined | Executed in the order they are defined (within the routes) |
Can be defined before or after the routes | Must be defined before the routes they are applied to |
Can have global and route-specific ordering | Can only have route-specific ordering |
Table D: Error Handling
Table D highlights the differences in error handling between App.All and App.Use middleware functions.
App.All Middleware | App.Use Middleware |
---|---|
Commonly used for global error handling | Error handling can be route-specific by invoking “next(err)” |
Allows catching errors for all routes in one place | Allows catching errors for specific routes separately |
Errors can be propagated to later middlewares | Errors can be propagated to later middlewares within the route |
Table E: Usage with Route Methods
Table E provides insights into how App.All and App.Use middleware functions are typically used with different route methods.
App.All Middleware | App.Use Middleware |
---|---|
Used with all route methods (GET, POST, PUT, DELETE, etc.) | Can be combined with specific route methods to create route-specific middlewares |
Executes for all routes, regardless of the route method | Executes only for routes using the defined route methods |
Effective for applying global middleware | Effective for applying route-specific middleware |
Table F: Reusability
Table F outlines the reusability aspect of App.All and App.Use middleware functions.
App.All Middleware | App.Use Middleware |
---|---|
Can be applied to multiple routes | Can also be applied to multiple routes |
Commonly used for global and generic middleware | Commonly used for route-specific middleware |
Offers flexibility in applying middleware across the application | Allows fine-grained control over middleware application |
Table G: Limitations
Table G highlights the limitations of both App.All and App.Use middleware functions.
App.All Middleware | App.Use Middleware |
---|---|
Cannot be applied to individual routes | Cannot modify the final response |
Cannot selectively execute for specific routes | Cannot access request/response globally |
May require additional route-specific middlewares for more control | Does not work effectively for global middleware |
Table H: Common Use Cases
Table H showcases some common use cases where either App.All or App.Use middleware functions are employed.
App.All Middleware | App.Use Middleware |
---|---|
Error handling middleware | Authentication middleware |
Request logging middleware | Authorization middleware |
CORS middleware | Data validation middleware |
Conclusion
In conclusion, while both App.All and App.Use middleware functions serve different purposes, they can be used together to create a powerful middleware pipeline. App.All middleware is handy for global error handling and general-purpose middleware, whereas App.Use middleware allows for precise control over route-specific middleware. By understanding the distinctions between these two middleware functions, developers can leverage them effectively to build robust and scalable web applications.
Frequently Asked Questions
What is the difference between App.All and App.Use?
App.All is a middleware that allows you to apply a middleware to all routes in your application, while App.Use is a middleware that applies to a specific route or a group of routes.
How can I use App.All in my application?
To use App.All, you simply call the App.All() method and pass in the middleware function as an argument. This middleware will then be applied to all routes in your application.
Can I use App.All multiple times in my application?
Yes, you can use App.All multiple times in your application. Each call to App.All will add the provided middleware to the existing middleware stack, allowing you to chain multiple middlewares together.
How can I use App.Use in my application?
To use App.Use, you call the App.Use() method and pass in the middleware function as an argument, along with the specific route or routes to which you want to apply the middleware. This middleware will then be applied only to the specified route(s).
Can I use App.Use without specifying a route?
Yes, you can use App.Use without specifying a route. In this case, the middleware will be applied to all routes in your application.
Is there a specific order in which App.All and App.Use should be used?
The order in which you use App.All and App.Use matters. App.All should be used before App.Use so that the middleware added using App.Use is applied after the middleware added using App.All.
Can I use both App.All and App.Use in my application?
Yes, you can use both App.All and App.Use in your application. App.All allows you to apply a middleware to all routes, while App.Use allows you to apply a middleware to specific routes. You can use them together to create a middleware stack with different levels of specificity.
Can I use App.All or App.Use multiple times for the same route?
Yes, you can use App.All or App.Use multiple times for the same route. Each call to App.All or App.Use will add the provided middleware to the existing middleware stack for that route, allowing you to chain multiple middlewares together for the same route.
What happens if there are conflicting middlewares added using App.All and App.Use?
If there are conflicting middlewares added using App.All and App.Use, the order in which they are added determines the execution order. The middleware added last will be executed first, followed by the middleware added earlier.
Can I remove a specific middleware from the middleware stack?
Yes, you can remove a specific middleware from the middleware stack by using the App.Remove() method. This allows you to selectively remove a middleware that was previously added using App.All or App.Use.